Querying using openCypher
PuppyGraph supports openCypher, the widely used implementation of the Cypher graph query language.
Graph Notebook
Graph Notebook is an open-source tool that enables users to interact with and visualize graph databases directly within a Jupyter Notebook environment.
PuppyGraph comes with a bundled Graph Notebook. To access it, click the Graph Notebook
tab on the Query
page. A new page will open with the Graph Notebook in it.
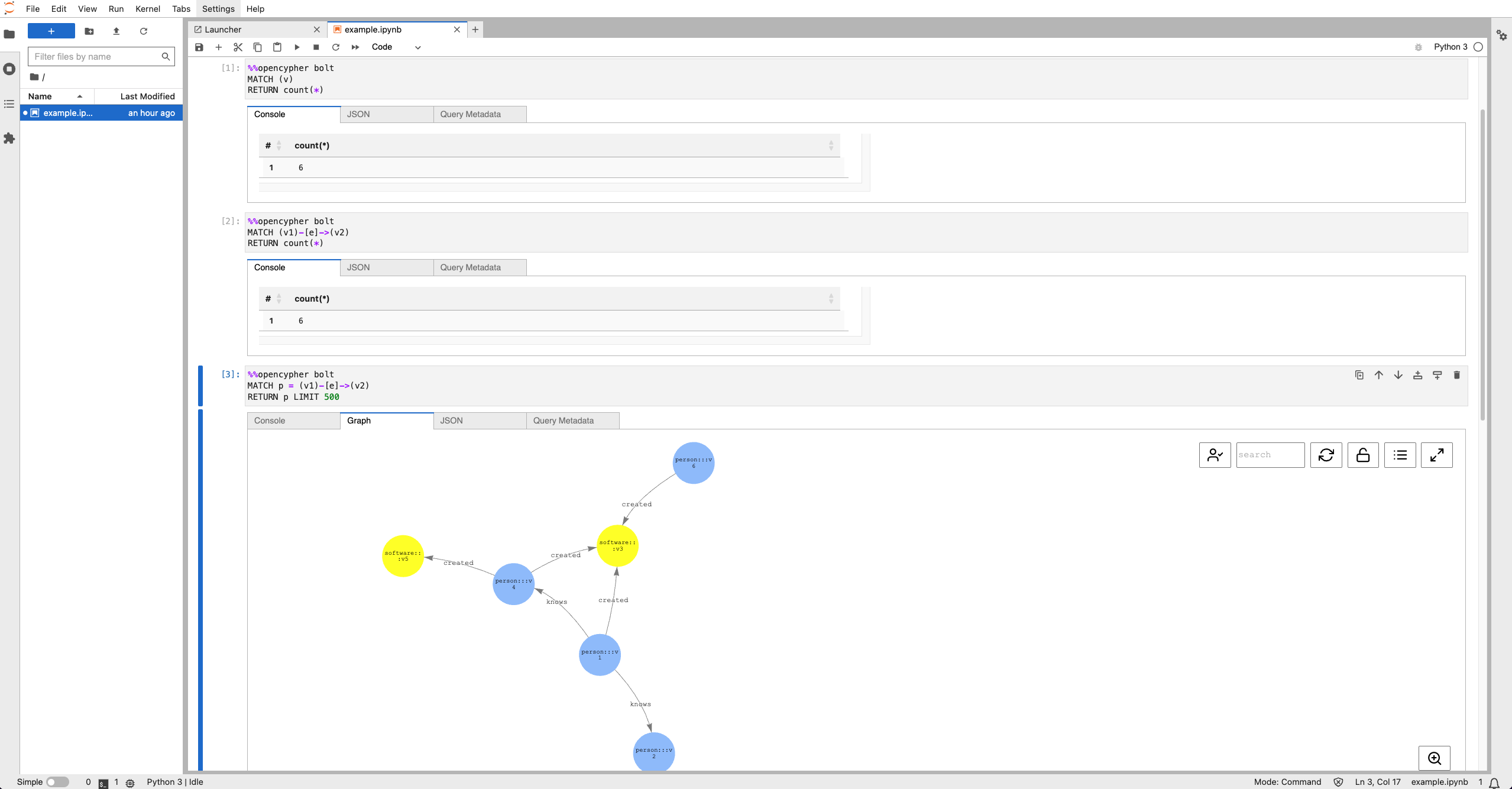
It comes with an example.ipynb
file with example queries.
Cypher Console
PuppyGraph provides an interactive command line, which is based on Gremlin Console and cypher-for-gremlin
plugin. To access it, click the Cypher Console
tab on the Query
page.
Info
Be sure to add:>
before the cypher query. See the screenshot below.
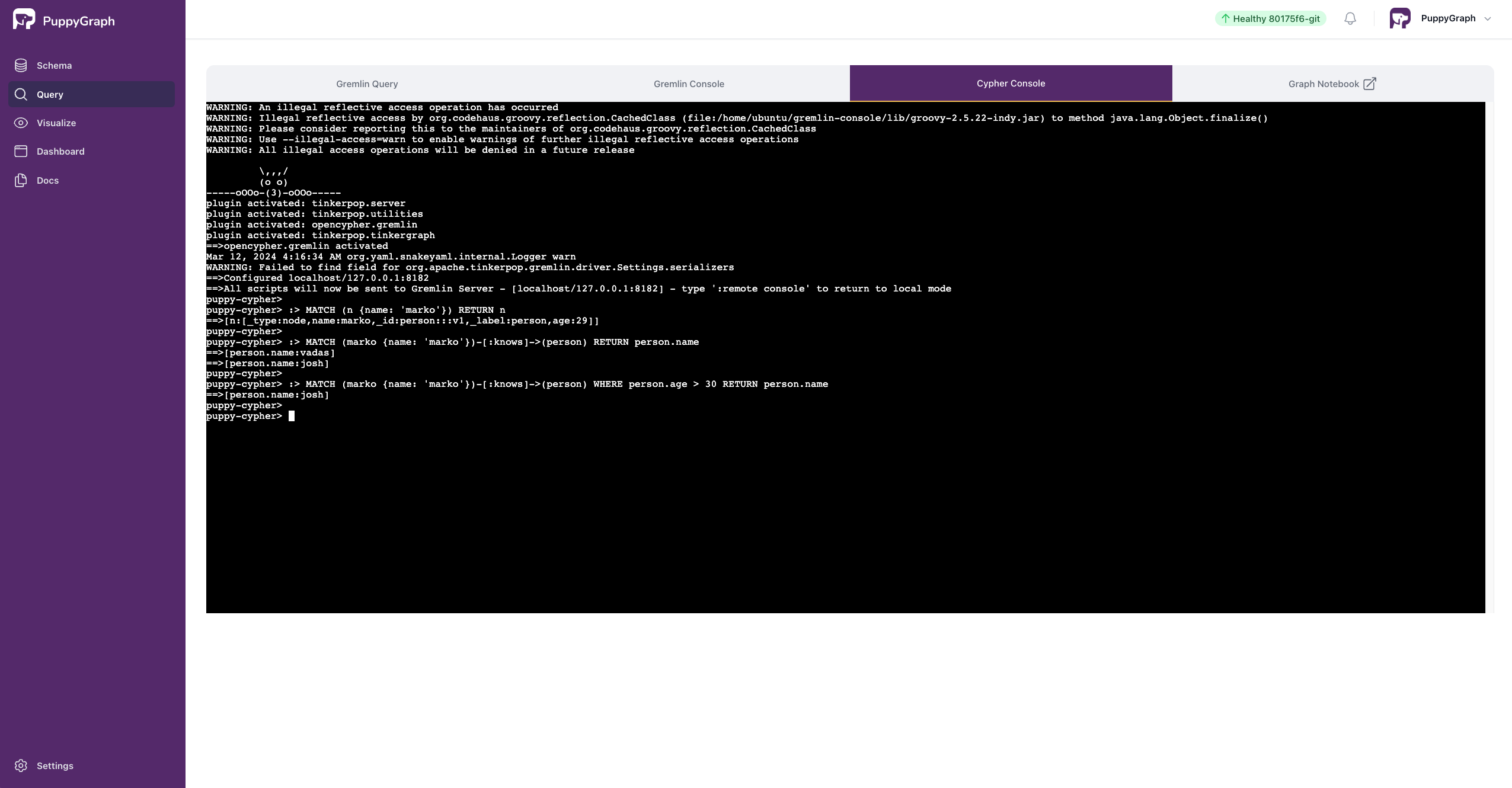
Client Drivers
PuppyGraph supports connecting and executing queries through the Bolt protocol of the following programming languages:
Python
Install the Neo4j Python Driver:
Here is an example of how to establish a connection to PuppyGraph and run a query:
from neo4j import GraphDatabase
# Initialize connection to the PuppyGraph.
uri = "bolt://localhost:7687"
username = "puppygraph"
password = "puppygraph123"
driver = GraphDatabase.driver(uri, auth=(username, password))
session = driver.session()
# Get all nodes from the graph.
query = "MATCH (n) RETURN n"
nodes = session.run(query)
print("All nodes in the graph:")
for record in nodes:
node = record["n"]
print({
"id": node.element_id,
"labels": list(node.labels),
"properties": dict(node._properties)
})
Go
Install the Neo4j Go Driver:
Here is an example of how to establish a connection to PuppyGraph and run a query:
package main
import (
"fmt"
"github.com/neo4j/neo4j-go-driver/v4/neo4j"
)
func main() {
// Creating the connection to the PuppyGraph.
uri := "bolt://localhost:7687"
username := "puppygraph"
password := "puppygraph123"
driver, err := neo4j.NewDriver(uri, neo4j.BasicAuth(username, password, ""))
if err != nil {
fmt.Println("Error creating driver:", err)
return
}
defer driver.Close()
// Open a new session using the driver
sessionConfig := neo4j.SessionConfig{AccessMode: neo4j.AccessModeRead}
session := driver.NewSession(sessionConfig)
defer session.Close()
// Get all the nodes (vertices) in the Graph.
query := "MATCH (n) RETURN n"
fmt.Println("All nodes (vertices) in the graph:")
results, err := session.Run(query, nil)
if err != nil {
fmt.Println("Error executing query:", err)
return
}
for results.Next() {
fmt.Println(results.Record().Values)
}
if err = results.Err(); err != nil {
fmt.Println("Error with query results:", err)
}
}
Java
Install the Neo4j Java Driver:
The example uses Maven. You need to add the following dependencies to the pom.xml
file of your project.
<dependency>
<groupId>org.neo4j.driver</groupId>
<artifactId>neo4j-java-driver</artifactId>
<version>4.4.3</version>
</dependency>
Here is an example of how to establish a connection to PuppyGraph and run a query:
import org.neo4j.driver.AuthTokens;
import org.neo4j.driver.Driver;
import org.neo4j.driver.GraphDatabase;
import org.neo4j.driver.Record;
import org.neo4j.driver.Session;
import org.neo4j.driver.Result;
import org.neo4j.driver.types.Node;
public class Example {
public static void main(String[] args) {
// Connect to PuppyGraph
Driver driver = GraphDatabase.driver("bolt://localhost:7687", AuthTokens.basic("puppygraph", "puppygraph123"));
Session session = driver.session();
// Get all the nodes (vertices) in the Graph.
Result result = session.run("MATCH (n) RETURN n");
System.out.println("All nodes (vertices) in the graph:");
while (result.hasNext()) {
Record record = result.next();
Node node = record.get("n").asNode();
System.out.println(String.format("Node ID: %s, Labels: %s, Properties: %s",
node.id(), node.labels(), node.asMap()));
}
// Close resources.
driver.close();
}
}
Javascript
Install the Neo4j JavaScript Driver:
Here is an example of how to establish a connection to PuppyGraph and run a query:
const neo4j = require('neo4j-driver');
const uri = 'bolt://localhost:7687';
const user = 'puppygraph';
const password = 'puppygraph123';
// Connect to PuppyGraph
const driver = neo4j.driver(uri, neo4j.auth.basic(user, password));
// Create a session to run Cypher statements in
const session = driver.session();
// Get all the nodes (vertices) in the Graph
const query = 'MATCH (n) RETURN n';
// Run the Cypher query
session.run(query)
.then(result => {
result.records.forEach(record => {
console.log(record.get('n'));
});
})
.catch(error => {
console.error('Error:', error);
})
.finally(() => {
return session.close(); // Close the session
})
.then(() => {
return driver.close(); // Close the driver connection
});